Introduction
|
- Various event listener interfaces are:
-
- ServletRequestListener Interface
- ServletRequestAttributeListener Interface
- ServletContextListener interface
- ServletContextAttributeListener Interface
- HttpSessionListener Interface
- HttpSessionAttributeListener Interface
- HttpSessionActivationListener Interface
The various events that are generated during the life cycle of a servlet are:-
- Servlet request events
- Servlet context events
- HTTP session events
Example for Implementing Servlet Life Cycle Events
CLICK HERE to download this complete example (zip file)
|
|
Create a Servlet that Adds Attributes to the Context Objects
|
-
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
import java.util.*;
public class ServletEvents extends HttpServlet
{
ServletContext ctx;
PrintWriter pw;
public void init(ServletConfig cfig)
{
ctx = cfig.getServletContext();
}
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException
{
ctx.setAttribute("URL","jdbc:odbc:EmployeesDB");
pw = response.getWriter();
response.setContentType("text/html");
pw.println("<B>The JDBC URL has been set as a context attribute at " + new Date() + "</B></BR>");
ctx.removeAttribute("URL");
pw.println("<B>The JDBC URL has been removed from context at " + new Date() + "</B>");
}
}
Download ServletEvents.java
|
Create a Servlet that Logs the Timer of Events
|
-
import javax.servlet.*;
import java.io.*;
import java.util.*;
public class ServletEventListener implements ServletContextListener, ServletContextAttributeListener, ServletRequestListener
{
ServletContext contx;
String name;
String value;
public void contextInitialized(ServletContextEvent ce)
{
contx=ce.getServletContext();
contx.log("Context has been initialized at " + new Date());
}
public void contextDestroyed(ServletContextEvent ce)
{
contx.log("Context has been destroyed at " + new Date());
}
public void attributeAdded(ServletContextAttributeEvent srae)
{
name=srae.getName();
value=(String)srae.getValue();
contx.log("An attribute with name: " + name + " and value: " + value + " has been added to the context at: " + new Date());
}
public void attributeRemoved(ServletContextAttributeEvent srae)
{
contx.log("Attribute with name: " + name + " and value: " + value + " has been removed from the context at: " + new Date());
}
public void attributeReplaced(ServletContextAttributeEvent srae)
{
contx.log("Attribute with name: " + name + " and value: " + value + " has been replaced context at: " + new Date());
}
public void requestInitialized(ServletRequestEvent e)
{
contx.log("A request has been initialized at: " + new Date());
}
public void requestDestroyed(ServletRequestEvent e)
{
contx.log("A request has been destroyed at: " + new Date());
}
}
Download ServletEventListener.java
|
Specify the initialization parameters
|
-
Write a java program and name it as ServletEvents.html
-
Write a java program and name it as ServletEventListener.java
-
Set the path in the command prompt
-
set path=.;C:\progra~1\java\j2sdk1.5.0\bin;C:\Sun\AppServer\bin;
-
Set classpath=.;C:\progra~1\java\j2sdk1.5.0\lib;C:\Sun\AppServer\lib\j2ee.jar;
(OR) Set the path in the system itself. CLICK HERE for details
-
Now compile the ServletEvents.java and ServletEventListener.java files. CLICK HERE to see how to compile
-
Goto Start->Programs->Sun Microsystems->Application Server PE->Start Default Server (Wait till it start and then press any key). CLICK HERE to see how to Start the Server
-
Goto Start->Programs-> Sun Microsystems->Application Server PE->Deploytool. CLICK HERE to see how to Start the Deploytool
- Goto File ->New -> Application Note: Inserted of EmployeeDetails use Listener
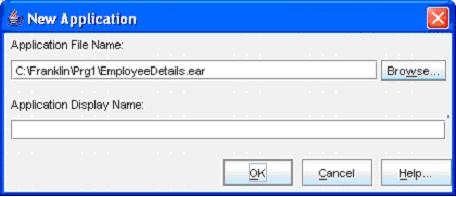 (Click the Browse button)
-
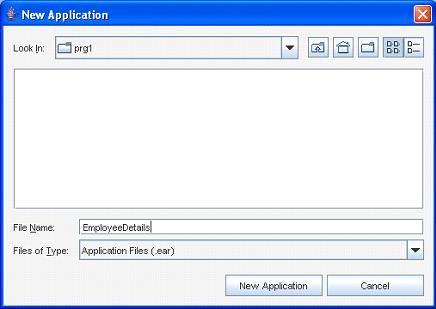 (Select the folder in the Look In dropdown box, and then give a file name “Listener”. Next click the New Application button)
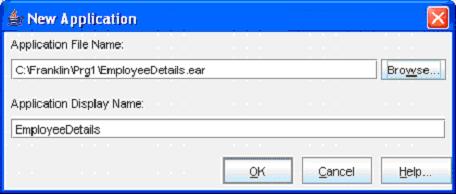 (Click the OK button)
- Now goto File -> Save to save the file
- Next, goto File -> New -> Web Component
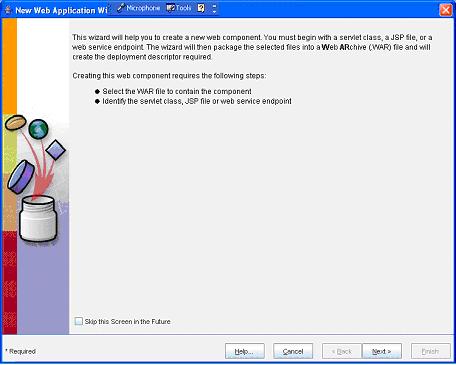 (Click Next button)
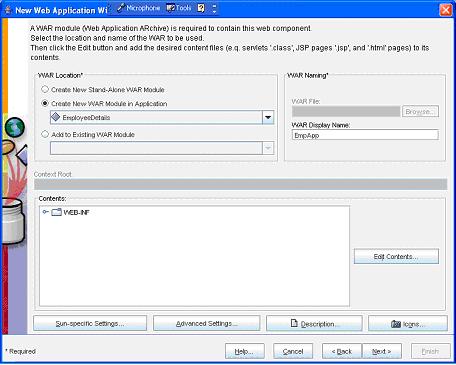 (Enter the WAR Name as “EmpApp” and then click the Edit Contents… button)
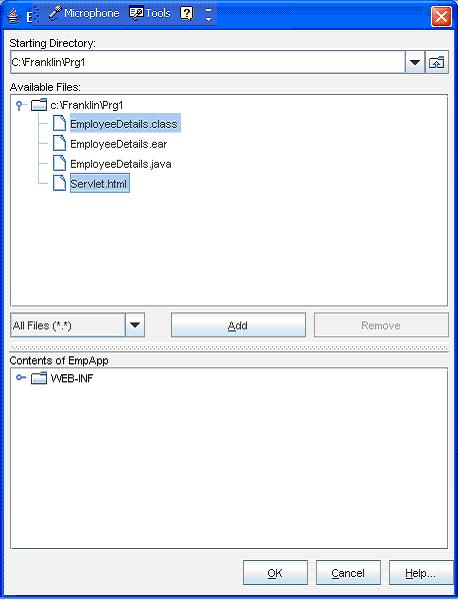 (Select all the .class, .jsp , .tld and .html files and click the Add button)
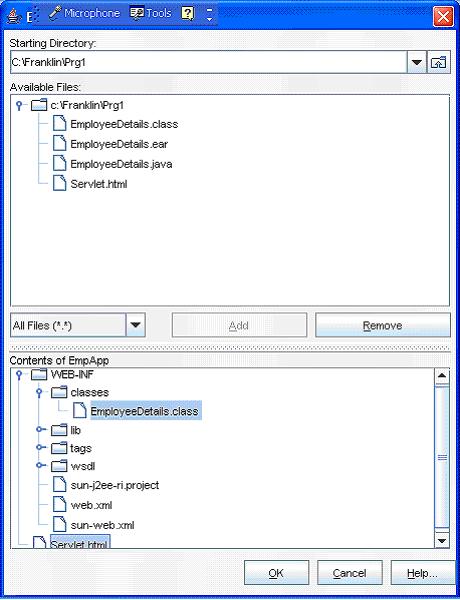 (Now click the OK button)
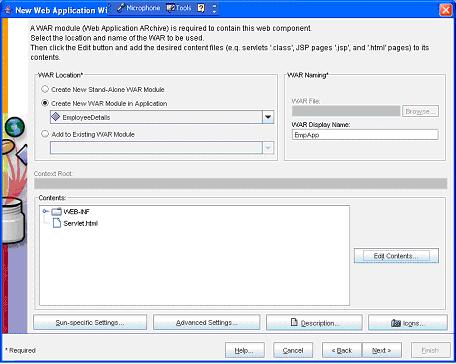 (Now click the Next button)
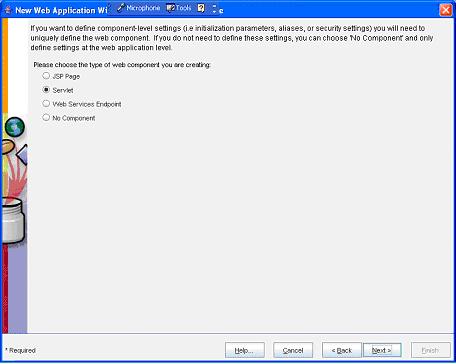 (Now select the Servlet option button and then click the Next button)
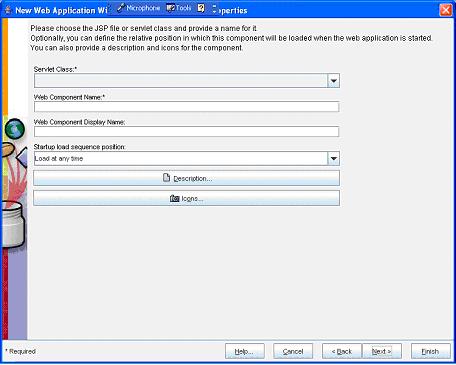 (Now select the “ServletEvents” from the Servlet Class dropdown box)
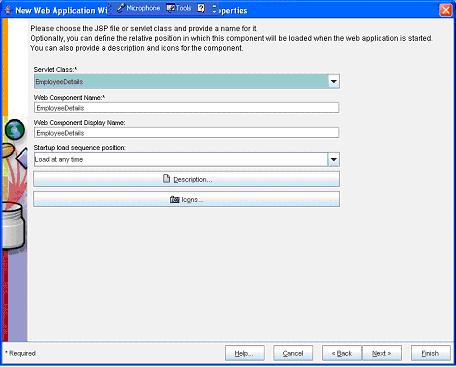 (Now select the Next button)
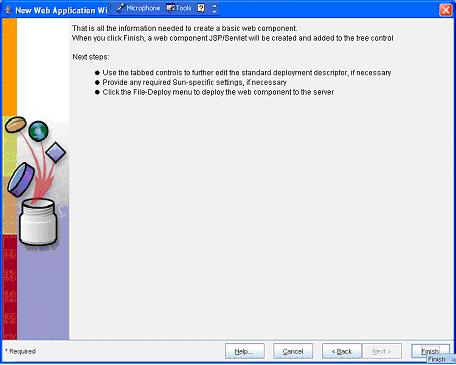 (Now select the Finish button)
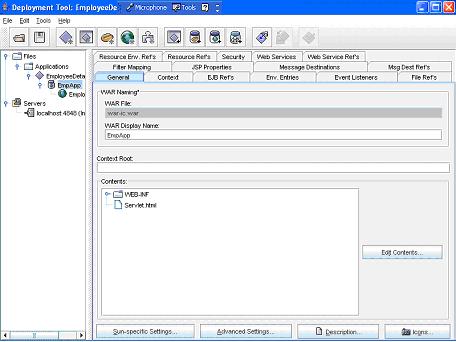 (Now select the EmpApp in the left pane and select the General tab in the right pane. Here give a name “example3” in the Context Root text box)
- Next select the InitParameterDemo in the right side
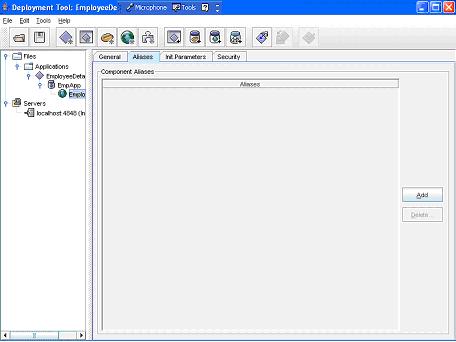 (Now select the ServletEvents in the left pane and then select the Aliases tab in the right pane. Next select the Add button)
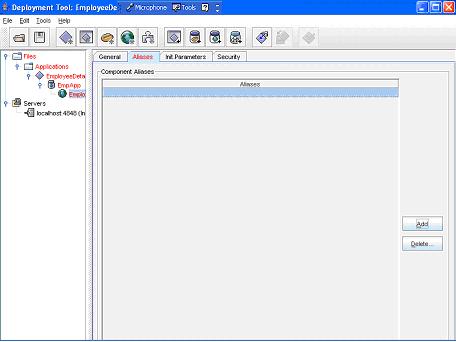 (Now add a name as “ServletEvents”)
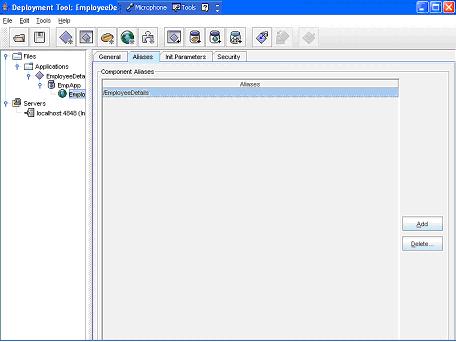
- Now select WebApp in the left pan and then select the Event Listeners tab in the right pane
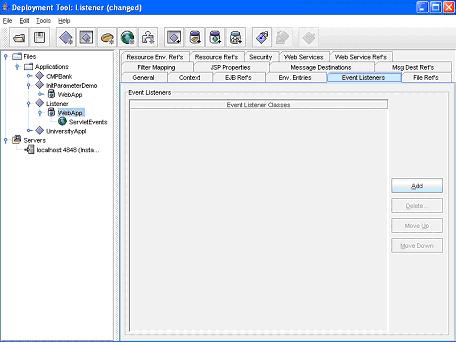
- Now click the Add button and then in the Event Listener Classes pane select the ServletEventListener
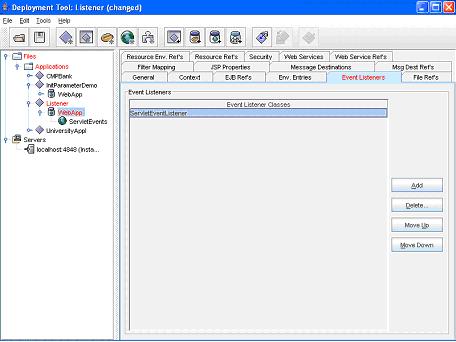
- Now goto File ->Save
- Next goto Tools -> Deployee
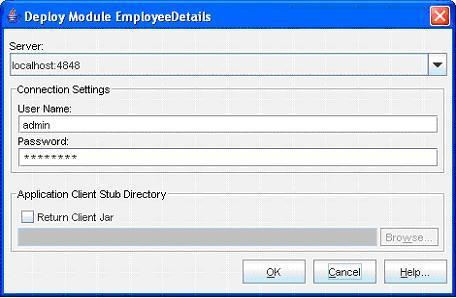 (Enter the User Name as “admin” and Password as “password” (CLICK HERE for password). Next click the OK button)
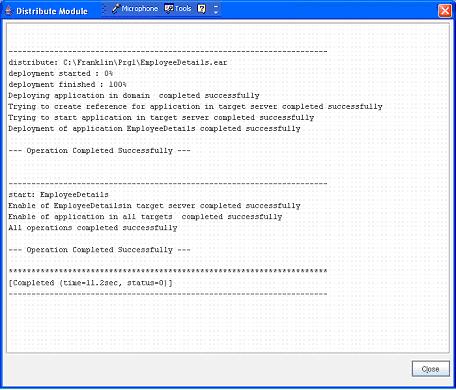 (Now a message --- Operation Completed Successfully --- must display. Next click the Close button)
- Next goto File -> Exit to close it
- Now open an Internet Explorer and type the address http:// localhost:8080/example3/ServletEvents
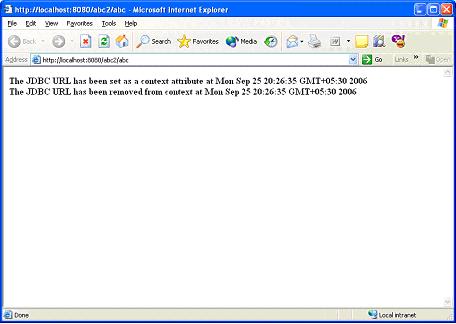
- The contents of the server.log file is shown in the figure (E:\Sun\AppServer\domains\domain1\logs\server.log)
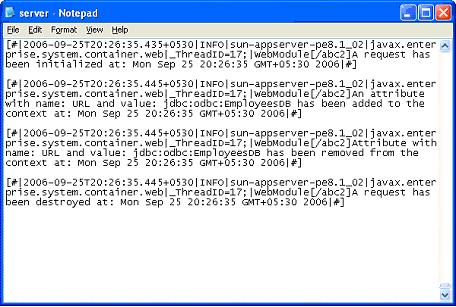
- Program completed Successfully
- To stop the server goto Start -> All Programs -> Sun Microsystems -> Application Server PE -> Stop Default Server. CLICK HERE to see how to Stop the Server
|
|
Click for Next Topic
|
|